For each sale, reprint or cancellation operation, the terminal will send the response in JSON format to a service so that it can be processed. Said service can be local on the intranet, or be publicly exposed on the internet through a URL or IP. The requirements for you to develop said service are detailed below.
7.1 Things to keep in mind.
- You can use any programming language you want and allow you to create a REST API.
- The service must be working whenever it is required to make payments with the terminal since that is where the response of the operation will be sent.
- The terminal must be able to reach the service at all times in order to perform the full flow of a transaction.
- In the event that the terminal indicates that it cannot send the response to the service, it must be verified immediately if the service is working correctly in order to carry out the correct flow of a transaction.
7.2 Requirements.
- When the service is consumed, it must return an HTTP Status Code 200.
- The returned HTTP verb must be POST.
- When consuming the service, the body must contain the JSON with the following parameters. (The message inside the message parameter can contain any text and the code parameter must return a 00).
{
"code": "00",
"message": "Recibido"
}
7.3 Data model
When a sale, reprint or cancellation is made, the response of the operation is sent in JSON format to the response service with a data model as shown in the following table:
Id | Field name | Description | Data type |
---|---|---|---|
1 | affiliation | Commerce affiliation number | VARCHAR2(50 BYTE) |
2 | applicationLabel | Information on card reading. | VARCHAR2(100 BYTE) |
3 | arqc | Information on card reading. | VARCHAR2(250 BYTE) |
4 | aid | Information on card reading. | VARCHAR2(50 BYTE) |
5 | amount | Total amount of transactions with everything and tip. | NUMBER(15,2) |
6 | authCode | Value generated by the authorization authority for an approved transaction. | VARCHAR2(7 BYTE) |
7 | bankName | Name of the financial institution issuing the card. | VARCHAR2(250 BYTE) |
8 | cardExpDate | Card expiration date in MM/YY format. | VARCHAR2(5 BYTE) |
9 | cardType | Card type identifier. Debit (D), credit (C). | VARCHAR2(20 BYTE) |
10 | cardTypeName | Represents the name of the card brand type, Visa, Master Card, etc. | VARCHAR2(250 BYTE) |
11 | cityName | City with which the trade was registered. | VARCHAR2(150 BYTE) |
12 | responseCode | Response code based on the status of the transaction. The value 00 represents a successful transaction, any other value can be taken as a problem with the transaction. | VARCHAR2(2 BYTE) |
13 | folioNumber | Transaction identification number that can be sent in the sale request. | VARCHAR2(250 BYTE) |
14 | hasPin | Indicates if the transaction was approved by PIN. | false o true |
15 | hexSign | Handwritten signature in case the card does not have a PIN. | VARCHAR2(4000 BYTE) |
16 | isQps | Indicates the amount charged by quick payment service. | VARCHAR2(1 BYTE) |
17 | message | Message indicating the status of the transaction. You can indicate if it was accepted or declined and for what reason. | VARCHAR2(200 BYTE) |
18 | moduleCharge | Internal identifier. | VARCHAR2(20 BYTE) |
19 | moduleLote | Internal identifier. | NUMBER (9) |
20 | customerName | Name of the cardholder. | VARCHAR2(100 BYTE) |
21 | terminalId | Terminal serial number. | VARCHAR2(20 BYTE) |
22 | orderId | Transaction number identifier. It can be used later in reprint and cancellation. | VARCHAR2(36 BYTE) |
23 | preAuth | Pre-authorization. | NUMBER (9) |
24 | preStatus | Pre-authorization. | NUMBER(15,2) |
25 | promotion | Indicates the number of months without interest in which a transaction was partialized. | VARCHAR2(20 BYTE) |
26 | rePrintDate | Indicates the version of the application. | VARCHAR2(100 BYTE) |
27 | rePrintMark | Internal identifier. | VARCHAR2(100 BYTE) |
28 | reprintModule | Internal identifier. | VARCHAR2(1 BYTE) |
29 | cardNumber | Last 4 digits of the card read. | VARCHAR2(50 BYTE) |
30 | storeName | Name of the trade registered. | VARCHAR2(60 BYTE) |
31 | streetName | Registered business address. | VARCHAR2(150 BYTE) |
32 | ticketDate | Date and time of the transaction. | VARCHAR2(100 BYTE) |
33 | tipAmount | Indicate the amount for tip, if sent. | NUMBER |
34 | tipLessAmount | Indicates the amount less tip. | NUMBER(15,2) |
35 | transDate | Date and time of the transaction. | DATE |
36 | transType | Indicates the type of operation performed. Sale (A), Cancellation (V). | VARCHAR2(5 BYTE) |
37 | transactionCertificate | Information on card reading. | VARCHAR2(50 BYTE) |
38 | traceability | Object for sending additional information. | Object |
Note.
The field "hexSign" Autograph Signature, is a value that contains the encrypted digital signature of the client and is a value of up to (4000 bytes).
7.4 Answers
Succesful transaction
Example of a successful transaction without PIN.
{
"affiliation": "7389108",
"applicationLabel": "BANCOMER VISA",
"arqc": "5769022C62DAD4BD",
"aid": "A0000000032010",
"amount": "2.0",
"authCode": "222222",
"bankName": "BANCOMER",
"bin": "415231",
"cardExpDate": "11/20",
"cardType": "D",
"cardTypeName": "VISA",
"cityName": "Guadalupe NUEVO LEON",
"responseCode": "00",
"folioNumber": "12356",
"hasPin": true,
"hexSign": "",
"isQps": 0,
"isRePrint": false,
"message": "Transacción exitosa",
"moduleCharge": "3",
"moduleLote": "1",
"customerName": " ",
"terminalId": "1490293930",
"orderId": "210304143337-1490293930",
"preAuth": "0",
"preStatus": 0,
"promotion": "00",
"rePrintDate": "1.2.8_20210203",
"rePrintMark": "VISA",
"reprintModule": "C",
"cardNumber": "8610",
"storeName": "COMERCIALIZADORA DE MANGUERAS DE CHIHUAHUA SA DE CV",
"streetName": "AVE PABLO LIVAS 7200",
"ticketDate": "MAR. 04, 21 14:33:38 ",
"tipAmount": "0.0",
"tipLessAmount": "2.0",
"traceability": {
"idReferencia": "CD0000000000"
},
"transDate": "2021-03-04 14:33:37.CST",
"transType": "A",
"transactionCertificate": "8757257129B19003"
}
{
"affiliation": "7389108",
"applicationLabel": "VISA CREDITO",
"arqc": "F1648A419561ED25",
"aid": "A0000000031010",
"amount": "1.0",
"authCode": "222222",
"bankName": "BANORTE",
"bin": "493173",
"cardExpDate": "01/21",
"cardType": "C",
"cardTypeName": "VISA",
"cityName": "Guadalupe NUEVO LEON",
"responseCode": "00",
"folioNumber": "1235",
"hasPin": false,
"hexSign": "00000100000001DA0000009E000000040800031CFF02FF02D3043B653263359F0AF79846FE503D29AFED727442B52244782286DA831EE8FF02289E30401A72299B0CFF006A849ED2C985BC47C6BE0506A5C1F7CA627C2C8EA39C3A84EE4A0D89F36138FF02075BA8846842C4588866931840DD512B7A16193699D76B63D6D348B05E8469DCFF02FF02FF02FF02FF02FF02D4BB2780FF0202B49CFF02845CFF022662E975F74CCAD8B498B36DCBFA94152C63BBB2E21FF0F72C3974DF7B32669E4FCB0E1D240E596EE1132C9EFEFF02005E71604847C92BF0D8D60071A5B63B99160DAF4239A40B47988EE516FCFF021B5A8E2CE96342E49BF2D7712C32868B797A18B38583A6A2717CC6132AFF005486EC5EDF1B11AD80FF025620FF023B90FF024CFF020DFF021380FF02C980FF0215FF024EFF02F0D7FD3D75E66A137FBD090581BBCCF304BDF9DFFED9FB1D47C3412F5ACF0499DD37C3EB3B780062B4FA74EB5C83532E221CFA9DC194153F6E548EFF025247B5AE6EAE339E3EE758C3D0871234AB7286F871FDE44099D18B8EC8FF024F0FC24435FB120E140C41A17DF990DED7F691CE2B4DA75A9E3830FF02FF000B80FF024DFF0206FF02FC6080FF02FF02FF02FF02FF02FF008AD016B3DABE23E8B9A740B3B4560822F62C040F510B7C5916D1DDD06565D5C70EB0737C10DE0CC7BA0385B566BAA560A641EFE2BF40C6190CFF02533D6F7718D9E905BC6F4DBA31B0BBE0EEF6DB728E749A1CDA3EC2D2084B8FB810B77720FF02431A72D9126620FF02FF02FF02",
"isQps": 0,
"isRePrint": false,
"message": "Transacción exitosa",
"moduleCharge": "1",
"moduleLote": "1",
"customerName": "TREJO PECINA/AGUSTIN ",
"terminalId": "1490293930",
"orderId": "210304142730-1490293930",
"preAuth": "0",
"preStatus": 0,
"promotion": "00",
"rePrintDate": "1.2.8_20210203",
"rePrintMark": "VISA",
"reprintModule": "C",
"cardNumber": "8425",
"storeName": "COMERCIALIZADORA DE MANGUERAS DE CHIHUAHUA SA DE CV",
"streetName": "AVE PABLO LIVAS 7200",
"ticketDate": "MAR. 04, 21 14:27:31 ",
"tipAmount": "0.0",
"tipLessAmount": "1.0",
"traceability": {
"idReferencia": "CD0000000000"
},
"transDate": "2021-03-04 14:27:31.CST",
"transType": "A",
"transactionCertificate": "2E8834BB688B2202"
}
{
"affiliation": "7389108",
"applicationLabel": "VISA CREDITO",
"arqc": "F1648A419561ED25",
"aid": "A0000000031010",
"amount": "1",
"authCode": "222222",
"bankName": "BANORTE",
"bin": "",
"cardExpDate": "01/21",
"cardType": "C",
"cardTypeName": "VISA",
"cityName": "Guadalupe,NUEVO LEON",
"responseCode": "00",
"folioNumber": "1235",
"hexSign": "00000100000001DA0000009E000000040800031CFF02FF02D3043B653263359F0AF79846FE503D29AFED727442B52244782286DA831EE8FF02289E30401A72299B0CFF006A849ED2C985BC47C6BE0506A5C1F7CA627C2C8EA39C3A84EE4A0D89F36138FF02075BA8846842C4588866931840DD512B7A16193699D76B63D6D348B05E8469DCFF02FF02FF02FF02FF02FF02D4BB2780FF0202B49CFF02845CFF022662E975F74CCAD8B498B36DCBFA94152C63BBB2E21FF0F72C3974DF7B32669E4FCB0E1D240E596EE1132C9EFEFF02005E71604847C92BF0D8D60071A5B63B99160DAF4239A40B47988EE516FCFF021B5A8E2CE96342E49BF2D7712C32868B797A18B38583A6A2717CC6132AFF005486EC5EDF1B11AD80FF025620FF023B90FF024CFF020DFF021380FF02C980FF0215FF024EFF02F0D7FD3D75E66A137FBD090581BBCCF304BDF9DFFED9FB1D47C3412F5ACF0499DD37C3EB3B780062B4FA74EB5C83532E221CFA9DC194153F6E548EFF025247B5AE6EAE339E3EE758C3D0871234AB7286F871FDE44099D18B8EC8FF024F0FC24435FB120E140C41A17DF990DED7F691CE2B4DA75A9E3830FF02FF000B80FF024DFF0206FF02FC6080FF02FF02FF02FF02FF02FF008AD016B3DABE23E8B9A740B3B4560822F62C040F510B7C5916D1DDD06565D5C70EB0737C10DE0CC7BA0385B566BAA560A641EFE2BF40C6190CFF02533D6F7718D9E905BC6F4DBA31B0BBE0EEF6DB728E749A1CDA3EC2D2084B8FB810B77720FF02431A72D9126620FF02FF02FF02",
"internalNumber": "",
"isQps": 0,
"isRePrint": true,
"message": "Transaccion Valida",
"moduleCharge": "1",
"moduleLote": "1",
"customerName": "TREJO PECINA/AGUSTIN",
"terminalId": "1490293930",
"orderId": "210304142730-1490293930",
"preAuth": "0",
"preStatus": 0,
"promotion": "0",
"rePrintDate": "1.2.8_20210203",
"rePrintMark": "VISA",
"reprintModule": "C",
"cardNumber": "8425",
"storeName": "COMERCIALIZADORA DE MANGUERAS DE CHIHUAHUA SA DE CV",
"streetName": "AVE PABLO LIVAS 7200",
"tableId": "",
"ticketDate": "MAR. 04, 21 14:27:31",
"tipAmount": "0",
"tipLessAmount": "0",
"traceability": {},
"transDate": "2021-03-04 14:27:30.0",
"transType": "A",
"transactionCertificate": "F1648A419561ED25"
}
{
"affiliation": "7389108",
"applicationLabel": "VISA CREDITO",
"arqc": "F1648A419561ED25",
"aid": "A0000000031010",
"amount": "1.0",
"authCode": "222222",
"bankName": "BANORTE",
"bin": "",
"cardExpDate": "21/01",
"cardType": "C",
"cardTypeName": "VISA",
"cityName": "Guadalupe NUEVO LEON",
"responseCode": "00",
"folioNumber": "1235",
"hasPin": false,
"hexSign": "",
"isQps": 0,
"isRePrint": false,
"message": "Transacción exitosa",
"moduleCharge": "2",
"moduleLote": "1",
"customerName": "TREJO PECINA/AGUSTIN",
"terminalId": "1490293930",
"orderId": "210304142730-1490293930",
"preAuth": "0",
"preStatus": 0,
"promotion": "00",
"rePrintDate": "1.2.8_20210203",
"rePrintMark": "VISA",
"reprintModule": "C",
"cardNumber": "8425",
"storeName": "COMERCIALIZADORA DE MANGUERAS DE CHIHUAHUA SA DE CV",
"streetName": "AVE PABLO LIVAS 7200",
"ticketDate": "MAR. 04, 21 14:27:31",
"tipAmount": "null",
"tipLessAmount": "1.0",
"traceability": {},
"transDate": "2021-03-04 14:32:22.CST",
"transType": "V"
}
User Canceled / time out
In case of sending a request to the terminal and it is canceled from the terminal or the waiting time, which is around 1 minute, runs out, the following message will be sent in JSON format to the response service:
{
"responseCode": "02",
"hasPin": false,
"internalNumber": "",
"message": "Cancelado por el Usuario",
"tableId": "",
"traceability": {}
}
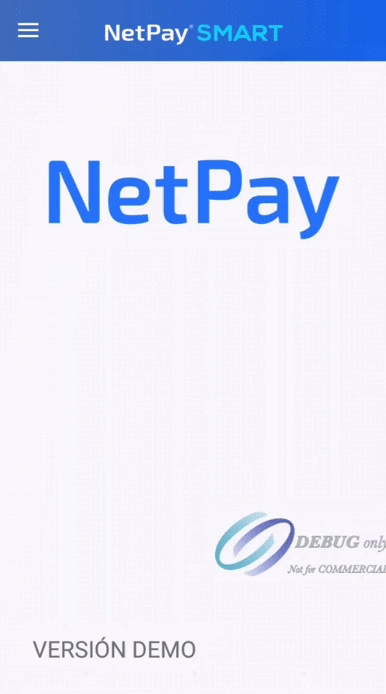
Demo venta Cancelado por el usuario.
Invalid promotion for card type
If a card is used for a certain type of promotion, such as months without interest, and it does not support said promotion (for example, a debit card), the following message will be received in JSON format:
{
"responseCode": "48",
"hasPin": false,
"internalNumber": "",
"message": "PROMOCION NO VALIDA PARA EL TIPO DE TARJETA",
"tableId": "",
"traceability": {}
}
Other types of responses
{
"responseCode": "13",
"hasPin": false,
"internalNumber": "",
"message": "TRANSACCION NO PERMITIDA",
"tableId": "",
"traceability": {}
}
{
"responseCode": "56",
"hasPin": false,
"internalNumber": "",
"message": "TARJETA INVALIDA",
"tableId": "",
"traceability": {}
}
{
"responseCode": "34",
"hasPin": false,
"internalNumber": "",
"message": "TARJETA RECHAZADA",
"tableId": "",
"traceability": {}
}
{
"bin": "",
"responseCode": "34",
"folioNumber": "prueba",
"internalNumber": "",
"isRePrint": false,
"message": "TRANSACCION RECHAZADA",
"tableId": "",
"traceability": {}
}
{
"bin": "",
"responseCode": "05",
"folioNumber": "prueba",
"internalNumber": "",
"isRePrint": false,
"message": "Error de conexión",
"tableId": "",
"traceability": {}
}
{
"bin": "",
"responseCode": "02",
"folioNumber": "prueba",
"internalNumber": "",
"isRePrint": false,
"message": "Cancelado por el Usuario",
"tableId": "",
"traceability": {}
}
As mentioned above, different types of response can be obtained depending on the card-issuing bank. A responseCode other than 00 indicates that the transaction was not successful and we can see the reason in the message.