Smart SDK.
The steps, to integrate your Android application with the NetPay SDK, are detailed below:
1. Add repository required.
The implementation of the SDK is through JitPack . This allow us to include remote repositories to our project.
The first step is to add Maven Jitpack to the list of repositories in the root gradle (Project) file.
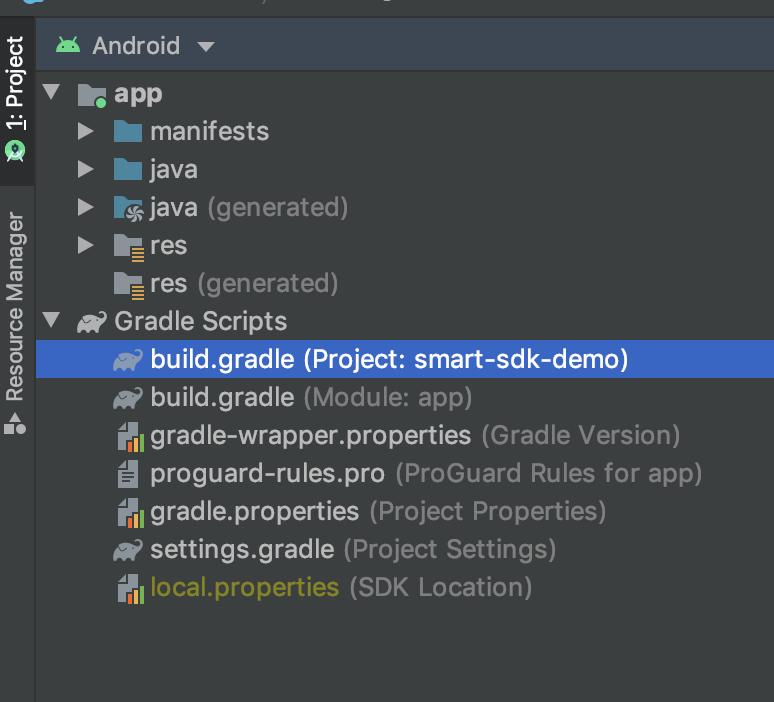
allprojects {
repositories {
...
maven { url 'https://jitpack.io' }
}
}
2. Add dependency information.
The next step is to add the dependency that you want to include on the application in the gradle file (Module: app).
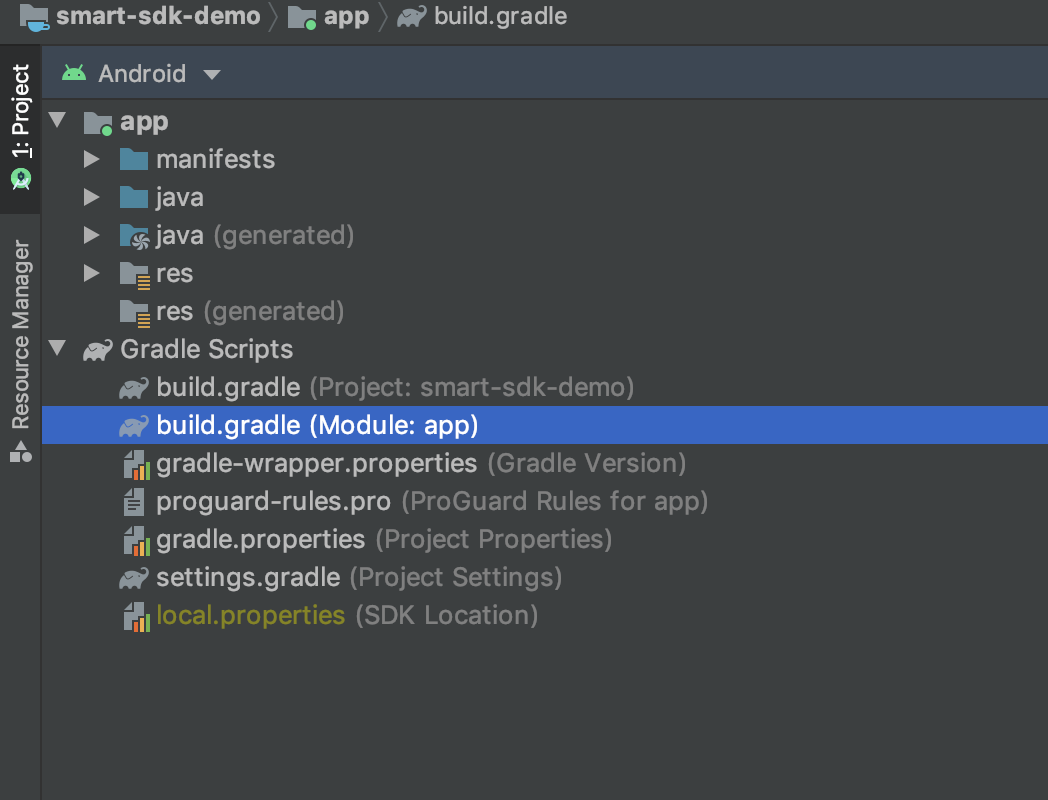
Note
You can check the latest released versions directly from Jitpack the most updated version is 1.1.5.
Example of how to add the dependency with version V1.1.5 is as follows.
dependencies {
implementation 'com.gitlab.netpaymx.netpay-sdks:smart-sdk:V1.1.5'
}
Demo SDK.
Note
In the following the drive link is attached a Demo SDK ready for download. https://drive.google.com/drive/folders/1nSUJ5yl8afwnyO4_LlmW-IjS4Sk0iOof
This link includes:
- APK ready to install DemoSDK-Oficial.apk".
- .zip folder with the Demo project "DemoSDK-2023.rar".
Demo SDK.
3. Import dependencies.
The following dependencies must be imported into your .java or .kt file where the sales operations will be used.
import mx.com.netpay.sdk.SmartApiFactory
import mx.com.netpay.sdk.exceptions.SmartApiException
import mx.com.netpay.sdk.models.*
4. Initialize the SDK.
You must initialize the SDK in order to make use of all available functionality by calling the createSmartApi() method.
In this example we create a constant called smartApi and assign the return value to it.
private val smartApi = SmartApiFactory.createSmartApi(this)
private SmartApi smartApi = SmartApiFactory.INSTANCE.createSmartApi(this);
5. Create SaleRequest object for a sale.
An instance of the SaleRequest class must be created that can receive the following parameters:
Parameter | Parameter type | Description. | Restrictions. |
---|---|---|---|
appId | String | Application package name. | *Mandatory |
amount | Double | Monto a cobrar, máximo 2 decimales. | *Mandatory |
msi | Int | Indicates the number of months to defer the purchase (3, 6, 9, 12, 18). | Optional |
tip | Double | Optional amount for tip, maximum 2 decimals. | Optional |
folio | String | Transaction identification number that can be sent in the sale request. | *Mandatory |
val sale = SaleRequest("mx.com.netpay.demosdk", amount,msi,tip,folio)
SaleRequest sale = new SaleRequest("mx.com.netpay.demosdk", amount, tip,msi, null, null,
null, null, folio, null, null, null, null,
null, null);
5.1 Implement tipping.
In case of implementing sales with tips, use the following code:
saleButton.setOnClickListener {
val folio = folio.text.toString()
val amount = amountTE.text.toString().toDoubleOrNull()?:0.0
val tip = tip.text.toString().toDoubleOrNull()?:0.0
val msi = msi.text.toString().toIntOrNull()()?:0
val sale = SaleRequest("mx.com.netpay.demosdk", amount, folio = folio,msi = msi, tip = tip)
try {
smartApi.doTrans(sale)
} catch (e: SmartApiException) {
Toast.makeText(this, e.message,Toast.LENGTH_LONG).show()
}
}
double amount = Double.parseDouble(amountEt.getText().toString());
double tip =Double.parseDouble(amountEt.getText().toString());
SaleRequest sale = new SaleRequest("mx.com.netpay.demosdk", amount, tip,null, null, null, null, null, null, null, null, null, null,null, null);
Note
To activate the tip option on the Smart PinPad DEV, go to the [settings] section.
https://netpay.readme.io/docs/introducci%C3%B3n-7#configuraci%C3%B3n-de-terminal-optional
6. Create method doTrans() to send a sale.
We can send a request to make the payment through the doTrans() method, which receives the SaleRequest type object created in the previous step as a parameter.
saleButton.setOnClickListener {
val folio = folio.text.toString()
val amount = amountTE.text.toString().toDoubleOrNull()?:0.0
val tip = tip.text.toString().toDoubleOrNull()?:0.0
val msi = msi.text.toString().toIntOrNull()()?:0
val sale = SaleRequest("mx.com.netpay.demosdk", amount, folio = folio,msi = msi, tip = tip)
try {
smartApi.doTrans(sale)
} catch (e: SmartApiException) {
Toast.makeText(this, e.message,Toast.LENGTH_LONG).show()
}
}
double amount = Double.parseDouble(amountEt.getText().toString());
double tip =Double.parseDouble(amountEt.getText().toString());
SaleRequest sale = new SaleRequest("mx.com.netpay.demosdk", amount, tip,null, null, null,
null, null, null, null, null, null, null,
null, null);
smartApi.doTrans(sale);
7. Create ReprintRequest object for Ticket reprint.
In order to reprint the Ticket of a sale made, it is necessary to create an instance of the class
ReprintRequest which receives the following parameters:
Parameter | Parameter type | Description | Restrictions |
---|---|---|---|
appId | String | Application package name. | *Mandatory |
orderId | String | Number of the order to reprint. | *Mandatory |
val sale = ReprintRequest("mx.com.netpay.demosdk", orderId )
try {
smartApi.doTrans(sale)
} catch (e: SmartApiException) {
Toast.makeText(this, e.message,Toast.LENGTH_LONG).show()
}
String orderId = orderIdEt.getText().toString();
ReprintRequest reprintRequest = new ReprintRequest("mx.com.netpay.demosdk", orderId);
smartApi.doTrans(reprintRequest);
8. Create CancelRequest object to cancel a sale.
If you want to cancel a previously made sale, you need to create a CancelRequest type object, which receives the following as parameters:
Parameter | Parameter type | Description | Restrictions |
---|---|---|---|
appId | String | Application package name. | *Mandatory |
orderId | String | Number of the order to cancel. | *Mandatory |
val sale = CancelRequest("mx.com.netpay.demosdk", orderId )
try {
smartApi.doTrans(sale)
} catch (e: SmartApiException) {
Toast.makeText(this, e.message,Toast.LENGTH_LONG).show()
}
String orderId = orderIdEt.getText().toString();
CancelRequest voidRequest = new CancelRequest("mx.com.netpay.demosdk", orderId);
smartApi.doTrans(voidRequest);
Note.
You can only cancel a transaction made on the same day, and the cancellation must be requested before 20:00:00 p.m. Mexico City (GMT-5) time.
9. Get the answer.
When the user is done with the subsequent activity and returns, the system calls the activity's onActivityResult() method. This method includes three parameters:
• requestCode (Int) [required]: Request code that was passed to startActivityForResult().
• resultCode (Int) [required]: Result code specified by the second activity. The value will be RESULT_OK in case the transaction is successful. If not, the result will be RESULT_CANCELED, either because the user was removed or the operation failed for some reason.
• data (Intent) [required]: Intent that carries the result data.
override fun onActivityResult(requestCode: Int, resultCode: Int, data: Intent?) {
if(data != null) {
val response = when(requestCode) {
Constants.SALE_REQUEST -> smartApi.onResult(requestCode,resultCode,data) as SaleResponse
Constants.CANCEL_REQUEST -> smartApi.onResult(requestCode,resultCode,data) as CancelResponse
Constants.REPRINT_REQUEST-> smartApi.onResult(requestCode,resultCode,data) as ReprintResponse
else -> null
}
tvResponse.text = Gson().toJson(response) //TextView para mostrar la respuesta en formato JSON
}
}
@Override
protected void onActivityResult(int requestCode, int resultCode, @Nullable Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if(data != null) {
BaseResponse response = null;
switch (requestCode) {
case Constants.SALE_REQUEST:
response = (SaleResponse) smartApi.onResult(requestCode,resultCode,data);
break;
case Constants.CANCEL_REQUEST:
response = (CancelResponse) smartApi.onResult(requestCode,resultCode,data);
break;
case Constants.REPRINT_REQUEST:
response = (ReprintResponse) smartApi.onResult(requestCode,resultCode,data);
break;
case Constants.PRINT_REQUEST:
response = (PrintResponse) smartApi.onResult(requestCode,resultCode,data);
break;
}
if(response != null) {
responseTv.setText(new Gson().toJson(response));
}
}
}
[See details of the Sales, Reprint and Cancellation response JSON](https://docs.netpay.com.mx/docs/smart-sdk#12-json-de-respuesta-venta-reimpresi%C3%B3n-y -cancel%C3%B3n).
10. Create PrintRequest object for custom Ticket.
If it is required to implement the print request, the following code can be used:
print.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
//Create a Page
IPage page = smartApi.createPage();
//Create unit containing text and other formats
IPage.ILine.IUnit unit1 = page.createUnit();
unit1.setText("Texto 1");
unit1.setGravity(Gravity.START);
//2 or more units can be added to a line and it will split into columns
IPage.ILine.IUnit unit2 = page.createUnit();
unit2.setText("Texto 2");
unit2.setGravity(Gravity.END);
//A line is created and its units are added.
page.addLine().
addUnit(unit1).
addUnit(unit2);
//A new unit is created
IPage.ILine.IUnit unit3 = page.createUnit();
unit3.setText("Texto 3");
unit3.setGravity(Gravity.CENTER);
//A new line is created and the last unit is added
page.addLine().addUnit(unit3);
//A request of the type PrintRequest is created with the package name of the app and the page created
PrintRequest printRequest = new PrintRequest("mx.com.netpay.demosdk", page);
smartApi.doTrans(printRequest);
}
});
11. Customize the logo on the sales receipt.
Ticket example with an implemented logo.
val page = smartApi.createPage()
val bm = BitmapFactory.decodeResource(resources,R.drawable.logo_ticket)
smartApi.doTrans(sale,page,bm)
Note.
The size of the logo must have a maximum height of 100px and a width of 150px. Otherwise it could cause conflict.
12. JSON response Sale, Reprint and Cancellation
{
"affiliation":" 9352287370",
"amount":"109.0",
"authCode":"222222",
"bin":"376665",
"cardType":"C",
"cardTypeName":"AMEX",
"folioNumber":"prueba1",
"isRePrint":false,
"orderId":"200917102306-0820649118",
"reprintModule":"C",
"spanRoute":"1234",
"transDate":"SEP,17,20 15:10:06",
"transType":"A",
"meessage":"Transaccion exitosa",
"success":true
}
{
"autCode":"222222",
"folioNumber":"prueba1",
"isRePrint":true,
"orderId":"200917102306-0820649118",
"reprintModule":"C",
"spanRoute":"8425",
"transType":"A",
"meessage":"Transaccion exitosa",
"success":true
}
{
"autCode":"222222",
"folioNumber":"prueba1",
"isRePrint":false,
"orderId":"200917102306-0820649118",
"reprintModule":"C",
"spanRoute":"8425",
"transType":"V",
"meessage":"Transaccion exitosa",
"success":true
}
Field | Description | Type | Example | Type of transactions to which it applies |
---|---|---|---|---|
success | If the answer is successful, answer with "true" if the answer is declined, answer with "false". | Boolean | Depending on the result: 1. """true"" 2. ""false""" | Sale. Reprint. Cancellation. |
message | Message indicating the status of the transaction. You can indicate if it was accepted or declined and for what reason. | String | Depending on the result: 1. """Successful transaction"" 2. ""Connection failed"" 3. ""TRANSACTION REJECTED" | Sale. Reprint. Cancellation. |
authCode | Value generated by the authorization authority for an approved transaction. | String | "222222" | Sale. Reprint. Cancellation. |
folioNumber | Transaction identification number that can be sent in the sale request. | String | "Test1" | |
spanRoute | Last 4 digits of the card. | String | "1234" | Sale. |
orderId | Transaction number identifier. It can be used later in reprint and cancellation. | String | "200917102306-0820649118" | Sale. Reprint. Cancellation. |
folioNumber | Transaction identification number that can be sent in the sale request. | String | Currently not sent in the return response | Sale. |
bin | Card bin First 6 digits of the card. | String | "376664" | Sale. |
amount | Total amount of the transaction with everything and tip. | String | "109.0" | Sale. |
cardTypeName | Represents the name of the card brand type, Visa, Master Card, etc. | String | Depending on the card entered: 1. "AMEX" 2. VISA 3. MASTERCARD | Sale. |
affiliation | Business affiliation number. | String | "9352287370" | Sale |
transDate | Date and time of the transaction. | String | "SEP,17,20 15:10:06" | Sale |
cardType | Card type identifier. Debit (D), Credit (C). | String | "C" | Sale. Reprint. Cancellation. |
isRePrint | Value that indicates if the transaction is Reprint "true" and if it is Cancellation or Sale it is "false" | Boolean | Sale and cancellation: "false" Reprint: "true" | Sale. Reprint. Cancellation. |
transType | Value that indicates if the transaction is Reprint "true" and if it is Cancellation or Sale it is "false" | String | Sale: "A" Reprint: "A" Cancellation: "V" | Sale. Reprint. Cancellation. |
reprintModule | Internal identifier. | String | "C" | Sale. Reprint. Cancellation. |
13. Other answers
{
"bin": "",
"folioNumber": "",
"isRePrint": false,
"message": "TRANSACCION RECHAZADA",
"success": false
}
{
"bin": "",
"folioNumber": "",
"isRePrint": false,
"message": "Error de conexión",
"success": false
}
{
"bin": "",
"folioNumber": "",
"isRePrint": false,
"message": "Cancelado por el Usuario",
"success": false
}
{
"bin": "",
"folioNumber": "",
"isRePrint": false,
"message": "PROMOCION NO VALIDA PARA EL TIPO DE TARJETA",
"success": false
}
Updated 11 months ago